Authorization and Authentication
To use the DailyStory REST API you will first need an API token. To create an API token login to DailyStory and then go to Account Settings > API Tokens
:
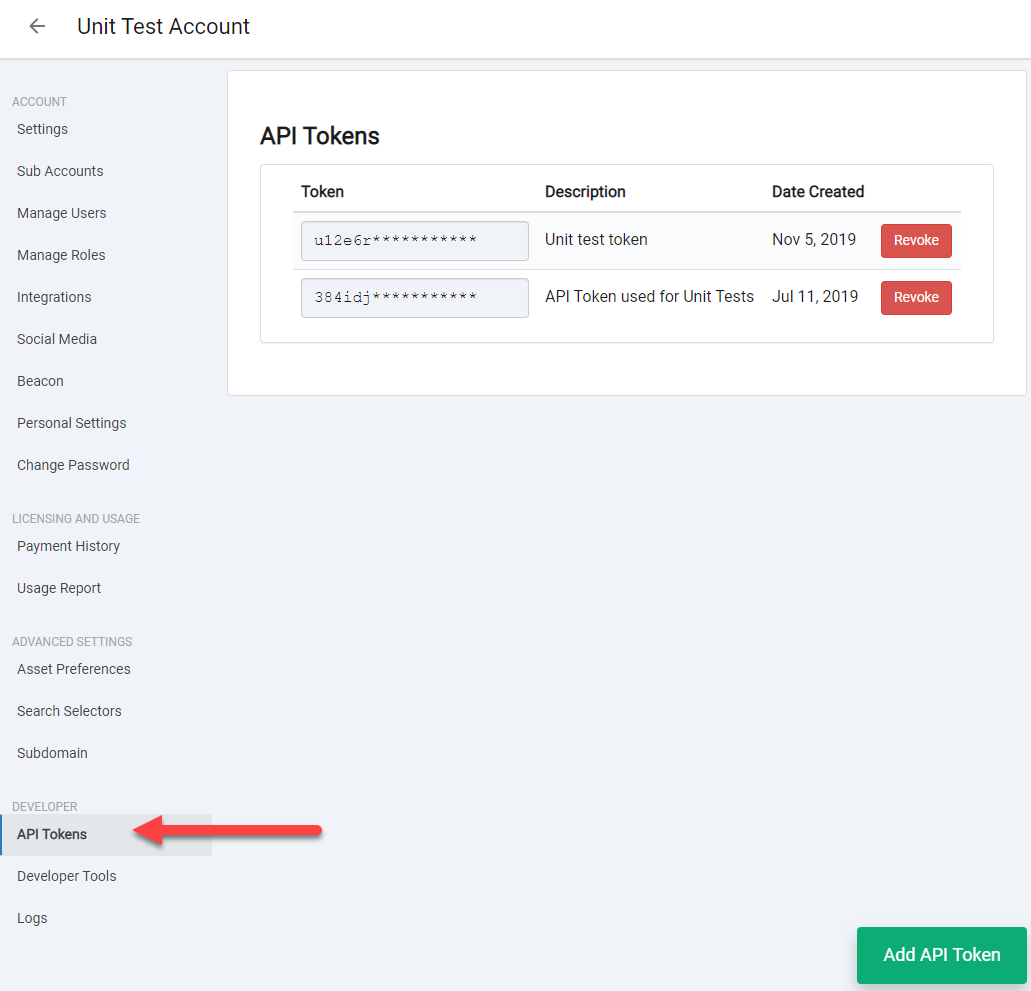
โFrom here you can click the "Add API Token" to generate a new token or revoke existing tokens.
Best Practice
As a best practice, we recommend creating separate tokens for each application integrated with DailyStory.
Using the API Token in a Request
All requests made to the DailyStory API require 2 pieces of information:
- API end point
- Request Authorization
API End Point
Use of the API requires you to use a specific data center identifier when making an API request. When logged in to DailyStory this can be determined by examining the URL.
For example, https://us-1.dailystory.com uses the us-1
data center. Whereas https://uk-3.dailystory.com uses the uk-3
data center.
Data Center required in the URL
All API requests are made using the URL that includes your data center, e.g. https://us-1.dailystory.com/api/v1/about
Request Authorization
The DailyStory API requires that all API requests are made over HTTPS and is authenticated using either basic authentication or a bearer token set in the Authorization HTTP header.
Basic Authentication
When using Basic Authentication the API token should be set as the password. The username can be set to any value, such as "api".
If you are creating a basic authentication HTTP Authorization header manually, don't forget to base 64 encode the username and password.
For example, given the following username and API token:
Username: api
API Token: some_secret_token
Format required for basic authentication before base 64 encoding:
api:some_secret_token
Base 64 encoded string:
YXBpOnNvbWVfc2VjcmV0X3Rva2Vu
The Authorization header for basic authentication would look as follows:
Authorization: Basic YXBpOnNvbWVfc2VjcmV0X3Rva2Vu
Bearer Token
Alternatively, you can also use the token as a bearer token. This is much simpler to work with. Simply set your Authorization header to "Bearer [your api token]".
You do not need to base 64 encoded the API token.
Given the following API token:
some_secret_token
The Authorization header for bearer authentication would look as follows:
Authorization: Bearer some_secret_token
GET request example
curl --user api:[YOUR API TOKEN] https://us-1.dailystory.com/api/v1/campaigns
curl -H "Authorization: Bearer {token}" https://us-1.dailystory.com/api/v1/campaigns
POST request example
curl -X POST --user api:[YOUR API TOKEN] -H "Content-Type: application/json" -d "{\"email\":\"[email protected]\"}" https://us-1.dailystory.com/api/v1/contact
curl -X POST -H "Authorization: Bearer {token}" -H "Content-Type: application/json" -d "{\"email\":\"[email protected]\"}" https://us-1.dailystory.com/api/v1/contact
API Response
The API will always respond with a JSON response. Even in the case of errors. Typically the API response will be wrapped in a Response object. For example, a request to the campaigns end-point (/api/v1/campaigns) returns:
{
"Status":true,
"Message":"",
"Response":{
"campaigns": [{...}]
}
}
The Status, along with the HTTP status code, indicates if the request was processed successfully. If an error occurred, the Message will contain details about the error.
The Response may contain multiple values.
Tips and Recommendations
Be sure and set the Content-Type to application/json in all POST requests.
Updated almost 3 years ago